Java is an option-oriented programming language. A programming language that helps its users develop a code to be written once and run on various platforms. These platforms must support Java and do not need any recompilation.
The developer of the Java Programming Language is James Gosling.
Key Takeaways
- A constructor is a special method called when an object is created, whereas a method is a function called on an object to perform a specific task.
- Constructors are used to initializing the object’s state, while methods perform actions on the object’s state or behavior.
- Constructors do not have a return type, while methods may or may not have a return type depending on their purpose.
Constructor vs Method
In OOPS (object-oriented programming), a constructor creates an instance of an object with desired attributes. It is implicitly invoked when an object is created. A method is a block of code that performs a specific task and can be called using the object reference. Methods can be invoked anytime during program execution. Constructors are only called during object creation.
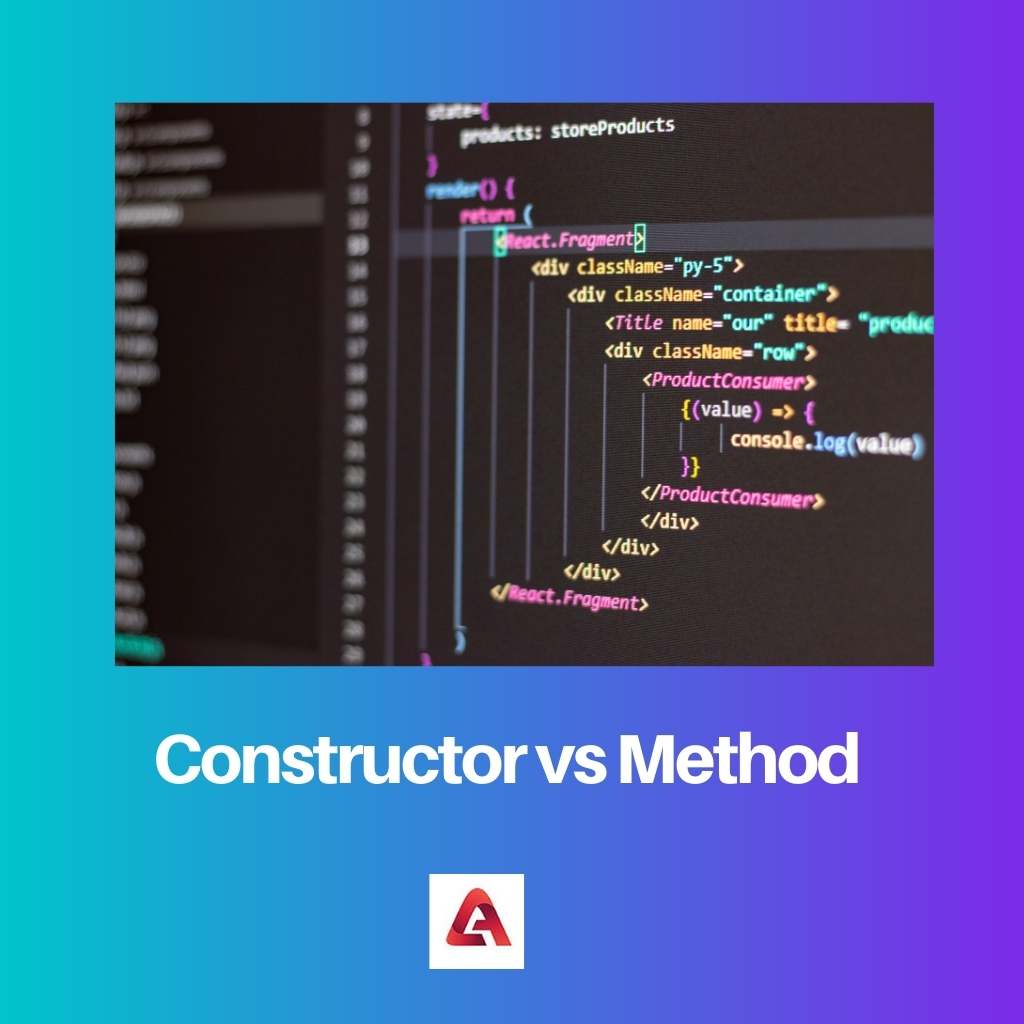
In object-oriented programming, a Constructor is a particular type of program instruction sequence that helps create a new object. It is said to be both explicit and implicit, i.e., it comes with parameterized constructor and no Arg constructor.
They also have an add-on feature that can be overridden.
The method is also object-oriented programming (OOP), which allows a specific sequence of programs associated with the message and the object. The method is said to be only explicit, which means it comes with a parameterized constructor.
It also entails statements that do not always return an output.
Comparison Table
Parameters of Comparison | Constructor | Method |
---|---|---|
Invocation | It is invoked implicitly by the system. | It is invoked during the program code. |
Uses | It is used to initialize an object. | It is used to exhibit the functionality of an object. |
Inheritance | A subclass cannot inherit it. | A subclass inherits it. |
Return Type | It does not have any return type. | It has a return type. |
Name | It is said that the constructor’s name must be the same as the class’s. | It is said that the name of the method cannot be the same as the class. |
What is Constructor?
A Java Constructor can be defined as an object-oriented program (OOP) with a specific series of program sequences that will help create a new object. It consists of both explicit (parameterized constructor) and implicit (no Arg constructor).
It can be overloaded but cannot be overridden. Also, it cannot be static, abstract, or real. The Constructor initializes an object and does not have any return type. It is said that the name of the constructor should be the same as the class.
For Example –
unit Test {
Test () {
// constructor body
}
}
In the above example, Test () is said to be the constructor. Thus, it proves the constructor has the same name and does not have any return type.
In the Java programming language, Constructors are of three types –
- No-Arg Constructor – It is said to be that Java Constructor may or may not have any parameters.
- Parameterized Constructors – The constructors which can accept one or more parameters are called Java Parameterized Constructors (constructors with parameters).
- Default Constructor – When no one creates any constructor, the Java compiler creates a constructor automatically while running a program. Thus, it is called a default constructor.
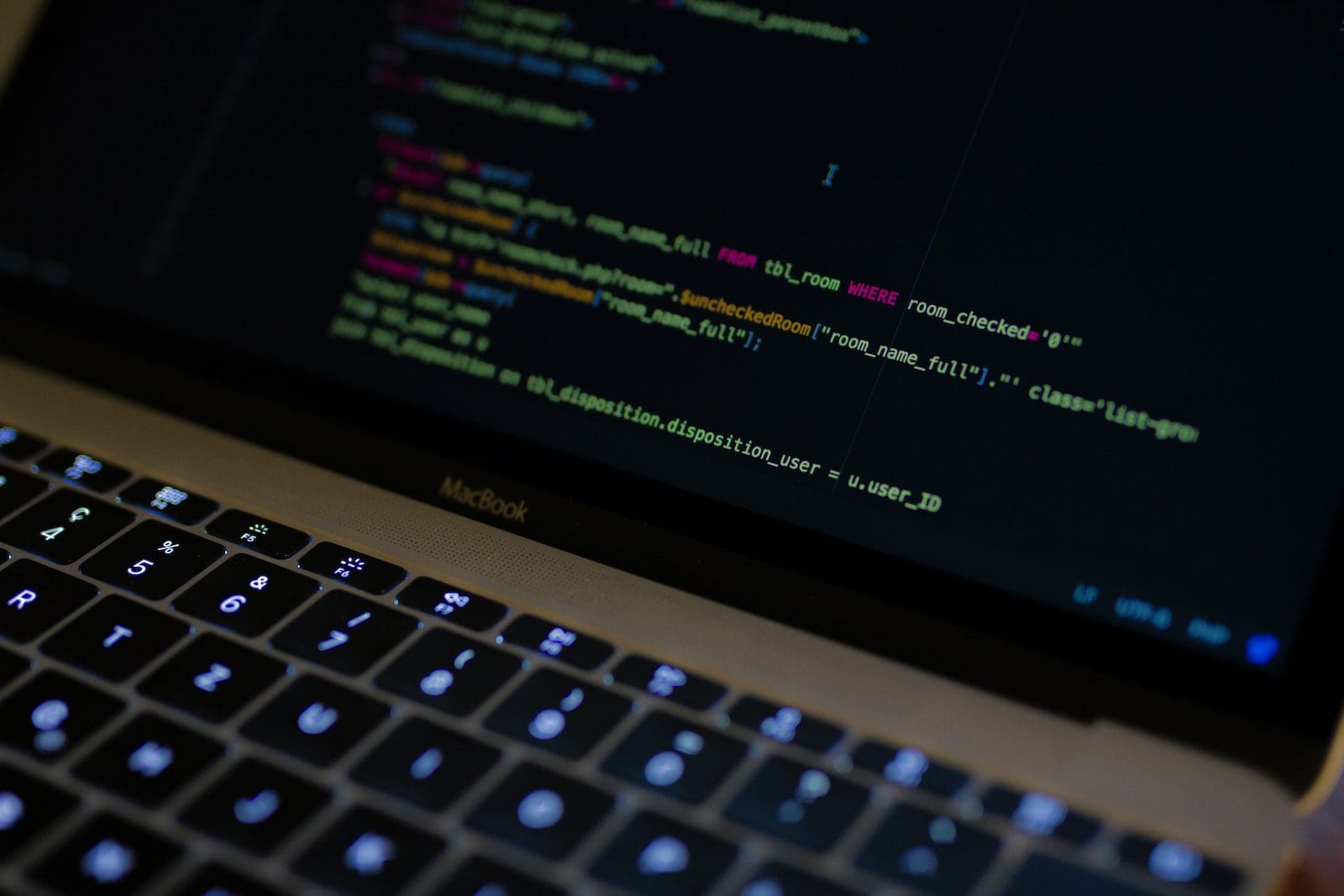
What is Method?
The Method is object-oriented programming (OOP) language that allows its user to run a specific series of programs associated with the message and the object. The Method is designed to perform only specific tasks and functions.
Unlike Constructor, it cannot have the same name as the constructor as the class. It also has a return type (including void). It is said to be that the Method should always be explicit (Parameterized Constructor).
Unlike Constructor, the Java compiler provides no default method if not created manually. Non-static methods are said to be inherited, and also, they can be overridden.
The method may use any object (non-static method), class reference (static method), or name. They also accept different parameter values. For Example –
A Burner has methods such as on or off, although the state of the burner, which is on or off, must be somewhat at a given time. This is known to be the property of the Burner.
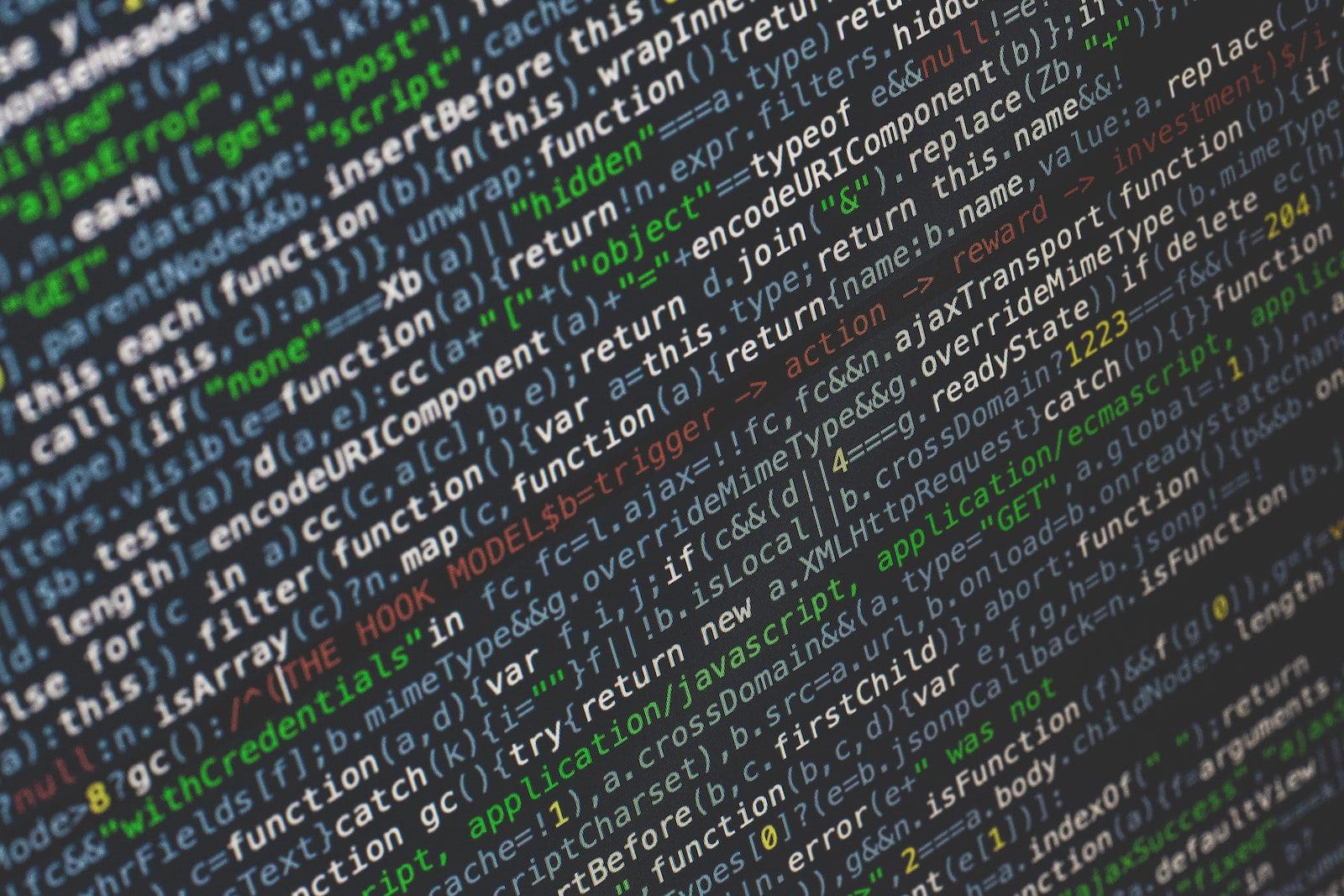
Main Differences Between Constructor and Method
- Constructor is said to be used implicitly by the system, while Method is used to invoke during the program code.
- Also, if there is an absence of a Constructor, then a default constructor can be taken into use provided by the Java compiler itself, while no default method can be used in case of its absence.
- Any subclass does not inherit a constructor, while a subclass can inherit Method.
- Constructors do not have any return type, while Method has a return type.
- While it is believed that the name of the Constructor must be the same as the class in contrast method.
- Constructors are said to be non-inherited, while contrastingly, non-static methods are said to be inherited.
- A Constructor can never be overridden, while a Method can be so.
- Java compiler provides a default constructor if you didn’t provide one but not a default Method.